配置环境
npm挂代理
- 查看npm配置信息
npm config list
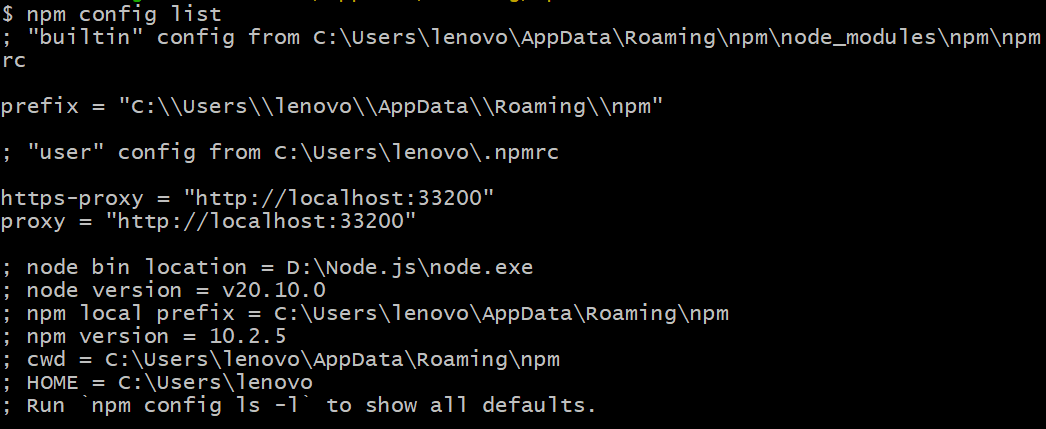
- 为npm设置代理
npm config set proxy http://server:port
npm config set https-proxy http://server:port
安装Git Bash
Git挂代理
Git配置文件位置
git config --list --show-origin
全局配置文件
局部配置文件
系统配置文件
设置和取消代理
git config --global https.proxy http://127.0.0.1:1080
git config --global https.proxy https://127.0.0.1:1080
git config --global --unset http.proxy
git config --global --unset https.proxy
安装Node.js
安装create-react-app
在终端执行npm i -g create-react-app
如果你遇到版本过低的警告又不想忽视他
请使用:
npx create-react-app create-react-app
cd create-react-app
npm start
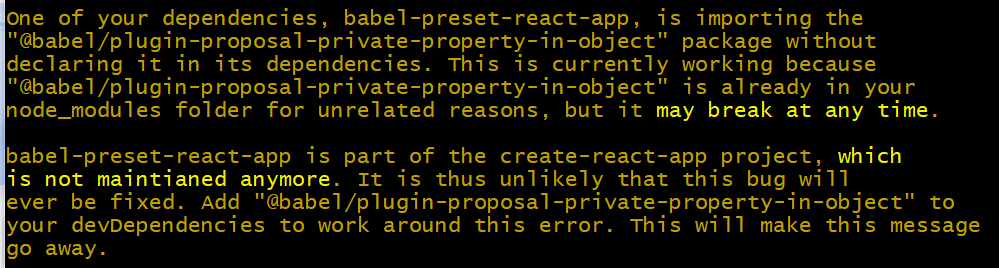
npm install --save-dev @babel/plugin-proposal-private-property-in-object
npm install --save-dev @babel/plugin-transform-private-property-in-object
安装vscode插件
Simple React Snippets
Prettier - Code formatter
创建React App
在你的项目目录下打开Git Bash或者你使用的终端
create-react-app react-app # 可以替换为其他app名称
cd react-app
npm start # 启动应用
vscode-powershell禁止运行脚本
如果你遇到如下错误:
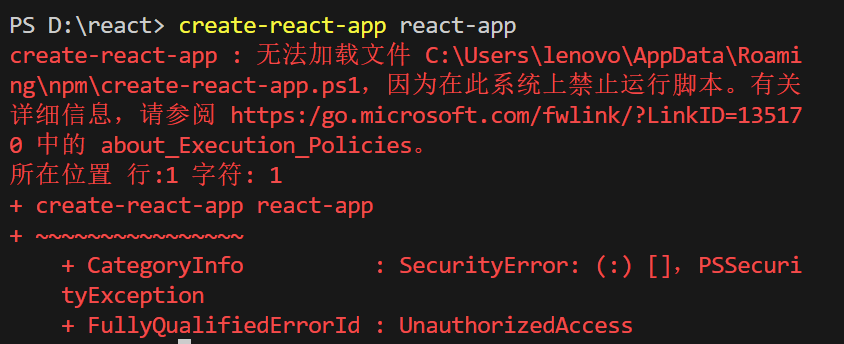
请使用git bash打开执行或者参考如下解决方案。
原因
PowerShell执行策略问题
解决方法
以管理员身份运行vscode;
执行:get-ExecutionPolicy,显示Restricted,表示状态是禁止的;

执行:set-ExecutionPolicy RemoteSigned;
这时再执行get-ExecutionPolicy,就显示RemoteSigned;
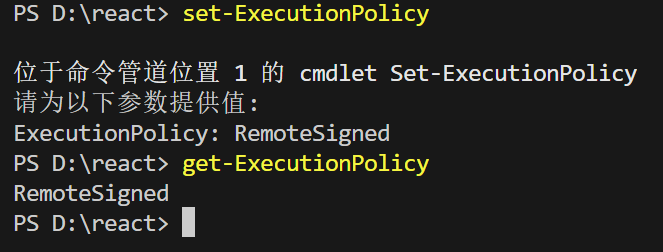
JSX && Babel
在JavaScript基础上,让我们能够支持xml。
Babel将jsx代码编译成js
ES6语法糖
使用bind()函数绑定this的值
const person = {
name: "yxc",
talk: function(){
console.log(this);
}
}
person.talk();
const talk = person.talk;
talk();
输出结果:

说明this输出的是运行时调用他的对象。
所有的全局变量挂在window下,所以第二个talk的this指向window
可以通过:
const talk = person.talk.bind(person);
将person这个变量绑定在talk这个函数上。
这样的运行结果是:

箭头函数简写方式
例如以下箭头函数:
const f = (x) => {
return x * x;
};
console.log(f(4));
如果输入只有一个参数,可以去掉参数括号:
const f = x => {
return x * x;
};
console.log(f(4));
当函数体只有一个return,可以删掉return,同时删掉大括号。
const f = x => x * x;
console.log(f(4));
箭头函数不重新绑定this
无箭头函数时:
const person = {
talk: function () {
setTimeout(function () {
console.log(this);
}, 1000);
},
};
person.talk();

使用箭头函数时:
const person = {
talk: function () {
setTimeout(() => {
console.log(this);
}, 1000);
},
};
person.talk();

因为箭头函数没有this,所以会向上找this。
对象的解构
传统写法:
const person = {
name: "yxc",
age: 18,
height: 180,
};
const name = person.name,
age = person.age;
console.log(name, age);
解构写法:
const person = {
name: "yxc",
age: 18,
height: 180,
};
const { name, age } = person;
console.log(name, age);
别名:
const person = {
name: "yxc",
age: 18,
height: 180,
};
const { name: new_name, age } = person;
console.log(new_name, age);
此时: new_name即为 name 的别名。
数组和对象的展开
let a = [1, 2, 3];
let b = [4, 5, 6];
let c = [-1,0,...a,3.5, ...b,7,8,9];
let a = [1, 2, 3];
let b = [4, 5, 6];
let c = [...a]; //此时a和c不是一个数组。深拷贝
let d = a; //此时a和d是一个数组。浅拷贝
if (c === a) {
console.log("a===c");
} else {
console.log("a!==c");
}
if (d === a) {
console.log("d===a");
} else {
console.log("d!==a");
}

字典的展开:
let a = { name: "yxc" };
let b = { age: 18 };
let c = { ...a, ...b, height: 180 };
console.log(c);
若声明对象,{“boxes”: “boxes”},若key和value一样,可以简写为{“boxes”}
Named与Default exports
- Named Export:可以export多个,import的时候需要加大括号,名称需要匹配
export default class Player {
constructor() {
console.log("new Player");
}
}
let id = 1;
export { id };
名字必须相同,但可以使用as定义别名
import MyPlayer, { id as id1 } from "./player";
new MyPlayer();
console.log(id1);
- Default Export:最多export一个,import的时候不需要加大括号,可以直接定义别名
export default class Player {
constructor() {
console.log("new Player");
}
}
引用的时候可以随意改名字,且只能创建一个
import MyPlayer from "./player";
new MyPlayer();
Component
新建项目
create-react-app box-app
安装bootstrap
cd ./box-app
npm i bootstrap
导入
import "bootstrap/dist/css/bootstrap.css";